rentx - car rental
I developed a full-stack web application for a car rental service, consisting of both frontend and backend systems. The backend was designed with a strong emphasis on clean architecture and domain-driven design (DDD) principles, while the frontend leverages modern web technologies like React and Next.js to deliver a smooth and responsive user experience.
The backend was built using Node.js with a strong focus on Domain-Driven Design (DDD) and Clean Architecture to ensure separation of concerns, scalability, and maintainability. Key features of the backend include:
- Clean Architecture & DDD: The application follows a modular structure, separating business logic from the infrastructure and delivery mechanisms. I used Aggregates and Watched Lists to maintain consistency across related objects and track changes effectively. This also allowed for easy scalability and extension of the application with additional subdomains and domain events.
- Functional Error Handling: I implemented a robust error-handling system based on functional programming techniques. This approach ensures that errors are caught and handled at appropriate layers without leaking across boundaries, improving the overall stability of the application.
- Use Case Tests: For each domain's core use cases, I developed comprehensive tests to ensure that all functionalities work as expected under various conditions. These tests are tightly coupled with the use case logic, offering high confidence in the behavior of the system.
- Layer Decoupling with NestJS: I used NestJS to decouple various layers of the application (e.g., domain, application, infrastructure). This decoupling made it easier to maintain and update individual components without affecting other parts of the system. NestJS controllers and services manage the application's API layer, while domain logic is handled independently.
- Domain Events & Internal Notifications: I implemented domain events to ensure that significant changes within the system (like car rental confirmations or cancellations) are appropriately handled across different services. Additionally, I developed an internal notification service to alert users about important updates within the system.
- Cache Integration: To optimize performance, I integrated caching mechanisms, ensuring that frequently requested data is stored and served quickly without overloading the database.
- Authentication: For security, I implemented JWT-based authentication, allowing users to securely log in using social login providers (Google, Facebook, etc.) or traditional email and password. This flexibility provides users with multiple ways to access their accounts.
- File Uploads & Relationships: I integrated file upload functionality, allowing users to upload relevant documents or images (e.g., driver’s license, vehicle images) as part of the rental process. Additionally, I managed complex relationships between entities, such as users, vehicles, and rental agreements.
- PostgreSQL & Docker: The backend uses PostgreSQL as the database, providing reliable and scalable data storage. The entire application runs within Docker, ensuring consistent environments for development, testing, and production. This also simplifies deployment across different platforms.
- Internal Notifications: I developed a custom service that handles internal notifications for users, making sure they stay informed about their car rental status or receive alerts for important system updates.
- Turborepo: The backend is structured as a monorepo using Turborepo, allowing me to manage multiple packages and services under a single repository. This approach simplified the development process by ensuring code reusability and efficient dependency management.
The frontend was developed using ReactJS (v19) in combination with Next.js (v15), providing a powerful foundation for building fast and dynamic web pages. Key features of the frontend include:
- ReactJS (v19): The frontend leverages the latest features of ReactJS, ensuring a modern, component-based architecture. This enables reusable UI components and efficient state management, which improves performance and maintainability.
- Next.js (v15): I used Next.js, a server-side rendering framework, which enhances the SEO of the car rental platform and allows for better performance by pre-rendering pages on the server before sending them to the client. This version of Next.js supports the use of Server Actions, improving the interaction between the frontend and the backend APIs.
- TailwindCSS: For styling, I used TailwindCSS, a utility-first CSS framework that allowed for fast and responsive design implementation. With Tailwind, I created a highly customizable and consistent UI design across all pages of the application, focusing on minimalism and user-friendliness.
- ShadCN UI: I integrated ShadCN UI components to create a modern and consistent interface, improving the user experience with interactive elements like car listings, filtering options, and booking forms. These UI components provided a professional look and feel, with smooth interactions and responsive design.
This project demonstrates my ability to build a scalable, secure, and user-friendly car rental application, from backend architecture to frontend implementation. By combining Node.js with NestJS and adhering to DDD principles, I ensured a maintainable and extensible backend, while ReactJS and Next.js provided the tools to deliver a seamless frontend experience. The use of technologies like TailwindCSS and ShadCN UI further enhanced the application's visual appeal and usability.
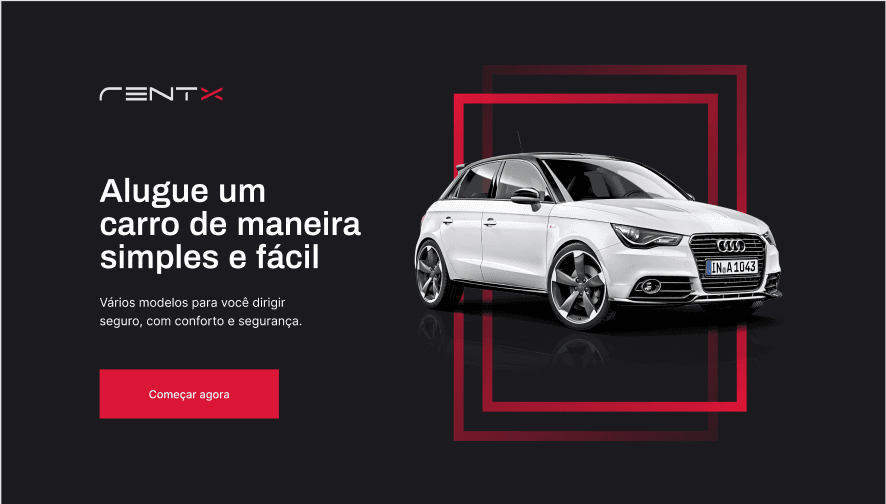